The Ultimate Guide to Error Handling in Python
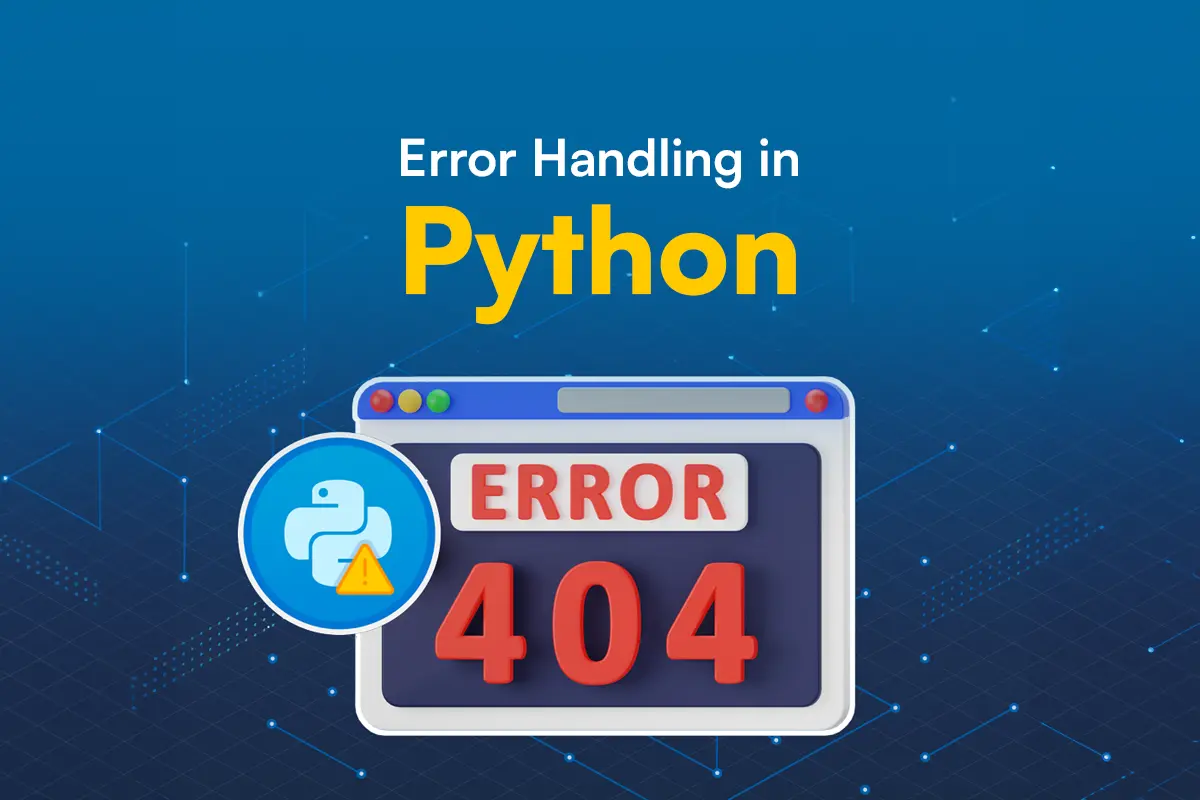
Introduction
Error handling in Python is like having a GPS for your code. Imagine you’re driving to a new destination, and suddenly, you miss a turn. Instead of ending your journey in frustration, your GPS calmly recalculates the route and gets you back on track. Error handling in Python works the same way—it helps your code recover from unexpected situations and continue running smoothly, ensuring that one wrong turn doesn’t disrupt your entire program.
In the world of Python development, error handling is crucial for ensuring robust, user-friendly applications. As errors are inevitable in any program, how we manage them can make the difference between a crash and a smooth user experience. This guide will walk you through Python’s error handling mechanisms, best practices, and advanced techniques to write clean, maintainable, and resilient Python code.
Types of Errors in Python
Syntax Errors
A syntax error in Python happens when the code violates the language’s structural rules, such as missing punctuation, misusing keywords, or incorrect indentation. These errors prevent the program from running and are immediately flagged by the interpreter, along with a message indicating the issue and its location.
Example:
File “c:\Users\name\OneDrive\Desktop\demo.py”, line 2 If x == 10 ^ SyntaxError: expected ‘:
Runtime Errors
Runtime errors occur during the execution of the program. These errors are caused by invalid operations or unexpected situations that arise when the code is running. Unlike syntax errors, which prevent the program from running at all, runtime errors allow the program to start but cause it to crash or behave unexpectedly while running.
Common Causes of Runtime Errors:
1. Division by Zero: This occurs when a division or modulo operation is performed with zero as the denominator.
Example:
2. Accessing Undefined Variables: This happens when a variable is referenced before it has been assigned a value.
Example:
print(a) # RuntimeError: name ‘a’ is not defined
3. Out-of-Bounds Indexing: When you try to access an element in a list or array using an invalid index, such as one that’s too large or negative.
Example:
4. File Not Found: If a file is attempted to be opened but it does not exist, it leads to a runtime error.
Example:
Logical Errors
Unlike syntax or runtime errors, logical errors do not cause the program to crash or throw an exception. These errors occur when the program runs successfully but produces incorrect or unexpected results due to flaws in the logic of the code. Logical errors are often the most difficult to identify and fix because the program behaves as though it is functioning properly, but the outcome is wrong.
Common Causes:
1. Incorrect Algorithm: Using the wrong formula or approach.
2. Off-by-One Error: A common mistake in loops or indexing.
3. Wrong Data Types: Misusing data types can lead to unexpected results.
4. Misplaced Conditions: Errors in conditionals can cause incorrect behavior.
Exceptions
In Python, exceptions are used to signal runtime errors that occur during the execution of a program. They allow the program to detect and handle unexpected conditions, such as invalid input or missing data, without crashing.
When an error occurs, Python raises an exception, which can be caught and managed using exception handling (via try, except blocks). This helps ensure that the program can recover gracefully or provide meaningful error messages to users.
Common Examples of Exceptions:
1. ValueError: Raised when a function receives an argument of the right type but inappropriate value.
int(“hello”) # ValueError: invalid literal for int() with base 10: ‘hello’
2. TypeError: Raised when an operation or function is applied to an object of inappropriate type.
“hello” + 5 # TypeError: can only concatenate str (not “int”) to str
3. KeyError: Raised when a dictionary key is not found.
How to Handle Exceptions:
Using a try block, you can attempt an operation that might cause an exception. If an exception is raised, it is caught in the except block, where you can handle it.
Example:
The Basics of Exception Handling
In Python, exception handling is a critical mechanism that ensures your program can handle runtime errors gracefully, without crashing unexpectedly. By using try, except, else, and finally blocks, Python offers a structured approach to catching and managing errors.
Basic Syntax of try and except
When you ask a user to input a number and divide 10 by it, this code handles errors like division by zero or invalid input (non-numeric).
Catching Multiple Exceptions
You can handle multiple exceptions using different except blocks or a single block for different errors.
Multiple except blocks:
Single except block:
Instead of separate error messages, one block handles both errors with a general message.
Using else and finally
else Block:
Executes if no exception is raised.
finally Block:
Ensures that cleanup code runs regardless of exceptions.
Built-in Python Exceptions
Python’s built-in exceptions provide an excellent mechanism for catching errors specific to the situation. By using the right exception handling, you can make your programs more robust, resilient, and user-friendly. Some of the common built-in exceptions include:
- ZeroDivisionError for division by zero.
- ValueError when a value is inappropriate for the operation.
- TypeError when an operation is applied to the wrong type.
- FileNotFoundError when a file is not found.
- IndexError for invalid list indices.
- KeyError for missing dictionary keys.
- AttributeError for invalid attribute references.
By understanding and using these built-in exceptions, you can create Python programs that can handle errors effectively and gracefully.
Raising Exceptions
In Python, exceptions can be raised explicitly using the raise keyword. This is important for cases when you want to signal an error manually, based on certain conditions in your code. Raising exceptions can make your program more robust, allowing you to handle issues more gracefully and provide users with clear error messages.
Let’s look at how to raise exceptions using both built-in and custom exceptions.
Raising Built-in Exceptions
There are scenarios where you need to raise a built-in exception, such as ZeroDivisionError or ValueError, to handle specific errors in your program. This is useful when you encounter an error condition that is common and needs to be handled properly.
Imagine you are developing a banking application that performs division for interest calculations. If the denominator (e.g., total balance) is zero, it would raise a ZeroDivisionError, ensuring that the program doesn’t crash and providing a meaningful message to the user. This helps maintain a smooth user experience in case of invalid input or erroneous data.
Creating Custom Exceptions
In cases where the built-in exceptions are not specific enough, you can create your own custom exceptions by subclassing the Exception class. This allows you to define application-specific errors and communicate them clearly to users, such as raising an InvalidAgeError for invalid age inputs in a registration system.
Example:
By raising exceptions intentionally, you can manage error handling in a more structured and clear way, ensuring that your code remains resilient and user-friendly even when unexpected conditions occur.
Best Practices for Error Handling
Effective error handling is key to writing reliable and maintainable code. Here are some best practices for handling exceptions in Python:
- Use Specific Exceptions
Catch only the specific exceptions you expect to occur. This makes your code clearer and ensures that you handle errors correctly. - Handle Only Expected Errors
Avoid suppressing unexpected errors. Let your program fail to detect issues early and address them. - Use else for Successful Execution
Use the else block to handle code that runs if no exceptions are raised. It separates normal code from error-handling logic. - Always Clean Up Resources with finally
Use the finally block for cleanup actions like closing files or releasing resources, ensuring they run regardless of exceptions. - Avoid Overusing Exception Handling
Don’t use exceptions for regular control flow. They should only handle exceptional cases, not routine logic. - Log Exceptions for Debugging
Log detailed exception information to help you diagnose issues and track them, especially in production environments. - Provide Meaningful Error Messages
Offer clear and informative error messages to users and developers to make it easier to understand and resolve issues.
By following these best practices, your code will be more robust, easier to maintain, and less prone to unexpected failures.
Error Handling in Real-World Scenarios
Effective error handling is essential in real-world applications, where various issues can arise. Here’s how to handle common scenarios:
1. Handling Database Errors
- Use Specific Exceptions: Database errors, such as connection failures or query issues, should be handled using specific exceptions like DatabaseError or IntegrityError.
- Transaction Management: Ensure transactions are rolled back in case of failures to maintain data integrity.
2. Handling File Operations
- FileNotFoundError: Catch this error when trying to access a non-existent file.
- PermissionError: Handle errors related to insufficient file access permissions.
- Resource Cleanup: Use finally or context managers (with statement) to ensure files are properly closed.
3. Handling APIs and Network Calls
- Timeout Errors: Handle timeouts by retrying or notifying the user if the request takes too long.
- Connection Errors: Catch network connection errors and provide fallback options or retries.
- API Response Errors: Handle specific HTTP errors (e.g., 400, 404, 500) to ensure appropriate responses and user feedback.
Tools for Debugging and Testing
- Debugging: Use pdb for interactive debugging.
- Testing: Write tests for error scenarios using pytest.
Conclusion
Mastering error handling in Python is essential for creating reliable and user-friendly applications. By understanding Python’s error handling mechanisms and following best practices, you can write code that gracefully handles unexpected situations, ensuring a seamless user experience.
Error handling is not just about fixing errors—it’s about writing code that’s robust, maintainable, and professional. Embrace these techniques, and take your Python skills to the next level!